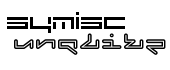
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
Jx9 Built-in Function - JSON Array & Object Processing.
int count($obj);
Alias
sizeof
Description
Counts all elements in an array, or total number of fields in an object.
Parameters
$obj: A JSON object or a JSON array.
Return Values
Returns the number of elements in $obj. 0 is returned on failure (i.e: scalar value).
Example
print count([10,11,12]); //3
$obj = { name: 'chems', age : 27 };
print count($obj); //2
Syntax
bool array_key_exists($key,$obj);
Description
array_key_exists() returns TRUE if the given key is a member of the target object.
Parameters
$key: Value to check (Any expression) .
$obj: A JSON object.
Return Values
Example
$obj = { name: 'dean', age : 27 };
print array_key_exists('name',$obj) ? 'Yes' : 'No' ; //Yes
value array_pop($array);
Description
Parameters
$array: A JSON array.
Return Values
int array_push($array,$val,...);
Description
Parameters
$array: A JSON array.
$val: Pushed value (Any expression).
Return Values
value array_shift($array);
Description
Parameters
$array: A JSON array.
Return Values
int64/float array_product($array);
Description
Parameters
$array: A JSON array.
Return Values
int64/float array_sum($array);
Description
Parameters
$array: A JSON array.
Return Values
array array_values($obj);
Description
Parameters
$obj: A JSON object or a JSON array.
Return Values
Example
$obj = { name: 'dean', age : 27 };
$arr = array_values($obj);
print $arr; //JSON array: ['dean',27]
bool array_same($obj1, $obj2);
Description
Parameters
$obj1: A JSON object or array.
$obj2: A JSON object or a JSON array.
Return Values
object array_merge($obj1,$obj2,...);
Description
Merges the elements of one or more arrays or object together so that the values of one are appended to the end of the previous one. It returns the resulting array or object.
Parameters
$obj1: A JSON object or a JSON array.
$obj2: A JSON object or a JSON array.
... Variable list of arrays or objects to merge.
Return Values
Example
$obj1 = { name: 'dean', age : 27 };
$obj2 = { address: '16 bla bla', phone : 55634257 };
$res = array_merge($obj1,$obj2);
print
$res; // JSON object { name: 'dean', age : 27 , address: '16 bla bla', phone : 55634257 }
array array_diff($obj1,$obj2,...);
Description
Compares obj1 against obj2 and returns the difference.
Parameters
$obj1: A JSON object or a JSON array.
$obj2: A JSON object or a JSON array.
... Variable list of arrays or objects to compare against.
Return Values
Returns an array containing all the entries from obj1 that are not present in any of the other objects.
Example
$obj1 = { name: 'dean', age : 27, phone : 97872916 };
$obj2 = { name : 'alex', age : 27, phone : 55634257 };
$res = array_diff($obj1,$obj2);
print $res; // JSON array [ 'dean' , 97872916 ]
Syntax
array array_intersect($obj1,$obj2,...);
Description
array_intersect() returns an array containing all the values of obj1 that are present in all the arguments. Note that keys are preserved.
Parameters
$obj1: A JSON object or a JSON array.
$obj2: A JSON object or a JSON array.
... Variable list of arrays or objects to compare.
Return Values
Returns an array containing all of the values in obj1 whose values exist in all of the parameters.
Example
$obj1 = { name: 'dean', age : 27, phone : 97872916 };
$obj2 = { name : 'alex', age : 27, phone : 55634257 };
$res = array_intersect($obj1,$obj2);
print $res; // JSON array [ 27 ]
Syntax
object array_copy($obj);
Description
Make a blind copy of the target object or array.
Parameters
$obj: A JSON object or a JSON array to copy.
Return Values
Copy of the target object or array on success. NULL otherwise.
Syntax
bool array_erase($obj);
Description
Remove all elements from a given object or array.
Parameters
$obj: A JSON object or a JSON array to copy.
Return Values
TRUE on success. FALSE otherwise.
Syntax
value key($obj);
Description
key() returns the index element of the current object or array position.
Parameters
$obj: A JSON object or a JSON array.
Return Values
The key() function simply returns the key of the object or array element that's currently being pointed to by the internal pointer. It does not move the pointer in any way. If the internal pointer points beyond the end of the elements list or the array is empty, key() returns NULL.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
while( $value = key($obj) ){
print "$value\n";
//Point to the next entry
next($obj);
}
//Should output:
//name
//age
//phone
Syntax
value current($obj);
Alias
Description
Return the current element in an array or object. Every object and array has an internal pointer to its "current" element, which is initialized to the first element inserted into the array.
Parameters
$obj: A JSON object or a JSON array.
Return Values
The current() function simply returns the value of the array element that's currently being pointed to by the internal pointer. It does not move the pointer in any way. If the internal pointer points beyond the end of the elements list or the array is empty, current() returns FALSE.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
while( $value = current($obj) ){
print "$value\n";
//Point to the next entry
next($obj);
}
//Should output:
//dean
//27
//97872916
Syntax
object each($obj);
Description
Return the current key and value pair from an object and advance the object cursor. After each() has executed, the object cursor will be left on the next element of the object, or past the last element if it hits the end of the object. You have to use reset() if you want to traverse the object again using each.
Parameters
$obj: A JSON object.
Return Values
Returns the current
key and value pair from the object obj.
This pair is returned in a four-element object, with the keys 0,
1,
key,
and value.
Elements 0
and key
contain the key name of the object element, and 1
and value
contain the data. If the internal pointer for the object points past
the end of the object contents, each()
returns FALSE.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
while( $pair = each($obj) ){
print $pair;
print JX9_EOL;
}
//Should output:
//{"1":"dean","value":"dean","2":"name","key":"name"}
//{"1":27,"value":27,"2":"age","key":"age"}
//{"1":97872916,"value":97872916,"2":"phone","key":"phone"}
Syntax
value next($obj);
Description
next() behaves like current(), with one difference. It advances the internal object pointer one place forward before returning the element value. That means it returns the next object value and advances the internal object pointer by one.
Parameters
$obj: A JSON object or a JSON array.
Return Values
Returns the object value in the next place that's pointed to by the internal object pointer, or FALSE if there are no more elements.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
while( $value = next($obj) ){
print "$value\n";
}
//Should output:
//27
//97872916
Syntax
value end($obj);
Description
end() advances object's internal pointer to the last element, and returns its value.
Parameters
$obj: A JSON object or a JSON array.
Return Values
Returns the value of the last element or FALSE for an empty object.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
print end($obj); //97872916
Syntax
value prev($obj);
Description
prev() behaves just like next(), except it rewinds the internal object pointer one place instead of advancing it.
Parameters
$obj: A JSON object or a JSON array.
Return Values
Returns the object value in the previous place that's pointed to by the internal object pointer, or FALSE if there are no more elements.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
end($obj); //Point to the next entry
while( $value = prev($obj) ){
print "$value\n";
}
//Should output:
//27
//dean
Syntax
value reset($obj);
Description
reset() rewinds object's internal pointer to the first element and returns the value of the first object element.
Parameters
$obj: A JSON object or a JSON array.
Return Values
Returns the value of the first object element, or FALSE if the object is empty.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
print reset($obj); //dean
Syntax
object array_map($callback,$obj);
Description
Applies the callback to the elements of the given object or array. array_map() returns an object containing all the elements of obj1 after applying the callback function to each one. The number of parameters that the callback function accepts should match the number of arrays passed to the array_map()
Parameters
$callback: Callback function to run for each element in each array.
$obj: A JSON object or a JSON array.
Return Values
Returns an array or object containing all the elements of obj after applying the callback function to each one.
Example
$arr = [ 12, 20, 60, rand() & 1023 ];
//A callback which return the given number multiplied by two
$func = function($num){
return $num * 2;
}; //Don't forget the semi-colon
//Apply our callback
print array_map($func,$arr); //[ 24, 40, 120, 440]
Syntax
bool array_walk($obj,$callback,$userdata);
Description
Applies the user-defined function ($callback) to each element of the given object or array. array_walk() is not affected by the internal object pointer. array_walk() will walk through the entire object or array regardless of pointer position.
Parameters
$obj: A JSON object or a JSON array.
$callback: Callback function to run for each element in each array. Typically, callback takes on two parameters. The object field value being the first, and the key/index second plus the optional userdata parameter.
$useredata: Optional user data passed as the third parameter to the callback.
Return Values
Returns TRUE on success or FALSE on failure.
Example
$obj = { name: 'dean', age : 27, phone : 97872916 };
//A callback which append a random string to the field value and output the result
$func = function($value,$key){
$value .= rand_str(3); //Random string of length 3
print "$value\n";
}; //Don't forget the semi-colon
//Invoke our callback
array_walk($obj,$func);
Syntax
bool sort($arr,[int $sort_type = SORT_REGULAR]);
Description
This function sorts an array. Elements will be arranged from lowest to highest when this function has completed.
Parameters
$arr: The input array.
$sort_type: Sorting type:
SORT_REGULAR - compare items normally (don't change types, default)
SORT_NUMERIC - compare items numerically
Return Values
Returns TRUE on success or FALSE on failure.
Example
$arr = [ 120, 70, 10-2, rand() & 1023 ];
sort($arr);
print $arr; //[8, 70, 120, 136]
Syntax
bool rsort($arr,[int $sort_type = SORT_REGULAR]);
Description
Sort an array in reverse order.
Parameters
$arr: The input array.
$sort_type: Sorting type:
SORT_REGULAR - compare items normally (don't change types, default)
SORT_NUMERIC - compare items numerically
Return Values
Returns TRUE on success or FALSE on failure.
Example
$arr = [ 70, 190, 5 * 3, rand() & 1023 ];
rsort($arr);
print $arr; //[877, 70, 190, 15]Syntax
bool usort($arr,$callback);
Description
Sort an array using an user supplied function as the comparison callback.
Parameters
$arr: The input array.
$callback: Comparison function. The comparison function must return an integer less than, equal to, or greater than zero if the first argument is considered to be respectively less than, equal to, or greater than the second.
Return Values
Returns TRUE on success or FALSE on failure.
Example
$arr = [ 70, 190, 5 * 3, rand() & 1023 ];
//Comparison function
$cmp = function($left,$right){
return $left > $right ? 1 : -1;
}; //Don't forget the semi-colon
usort($arr,$cmp);
print $arr; //[15 70, 101, 190]Syntax
bool in_array($needle,$obj,[bool strict = FALSE]);
Description
Searches haystack for needle using loose comparison unless strict is set.
Parameters
$needle: The searched value.
$arr: A JSON objet or a JSON array.
Return Values
Returns TRUE on success (Value found). FALSE otherwise.
Example
$arr = [ 'Win', 'OS X', 'FreeBSD', 'Linux' ];
if( in_array('FreeBSD',$arr) ){
print 'Got FreeBSD';
}
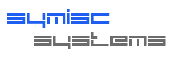