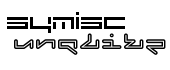
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
UnQLite C/C++ API Reference - Database Engine Handle.
Opening a new database connection.
Description
This routine allocate and initialize a new database object handle whose name is given by the zFilename argument. A database connection handle is usually returned in *ppDb but, if the engine is unable to allocate memory to hold the unqlite object, a NULL will be written into *ppDb instead of a pointer to the unqlite object. This routine is often the first API call that an application makes and is a prerequisite in order to work with the database library.
If zfilename is ":mem:" or NULL, then a private, in-memory database is created for the connection. The in-memory database will vanish when the database connection is closed. Future versions of UnQLite might make use of additional special filenames that begin with the ":" character. It is recommended that when a database filename actually does begin with a ":" character you should prefix the filename with a pathname such as "./" to avoid ambiguity.
Note: Transactions are not supported for in-memory databases.
The last parameter (iMode) control the database access mode (See parameter description for possible combination).
Note: This routine does not open the target database file. It merely initialize and prepare the database object handle for later usage.
Parameters
ppDb
|
OUT: A fresh database connection handle is written into this pointer. |
zFilename
|
Relative or full path to the target database file. If zfilename is ":mem:" or NULL then a private, in-memory database is created for the connection. |
iMode
|
Database access mode, the possible flag combination
is as follows: UNQLITE_OPEN_CREATE: If the database does not exists, it is created. Otherwise, it is opened with read+write privileges. This is the recommended access control flags for most applications.
UNQLITE_OPEN_READWRITE: Open the database with read+write privileges. If the database does not exists, an error code is returned.
UNQLITE_OPEN_READONLY: Open the database in a read-only mode. That is, you cannot perform a store, append, commit or rollback operations with this control flag.
UNQLITE_OPEN_MMAP: Obtain a read-only memory view of the whole database. This flag works only in conjunction with the UNQLITE_OPEN_READONLY control flags (i.e: unqlite_open(&pDb,"test.db",UNQLITE_OPEN_READONLY|UNQLITE_OPEN_MMAP); ). You will get significant performance improvements with this combination but your database is still read-only.
UNQLITE_OPEN_TEMP: A private, temporary on-disk database will be created. This private database will be automatically deleted as soon as the database connection is closed.
UNQLITE_OPEN_MEM: A private, in-memory database will be created. The in-memory database will vanish when the database connection is closed.
UNQLITE_OPEN_OMIT_JOURNALING: (Not recommended) Disable journaling for this database. In other words, you will not be able to rollback your database after a crash or power failure. This flag is automatically set for temporary database.
UNQLITE_OPEN_NO_MUTEX: (Not recommended) Disable the private recursive mutex associated with each database handle. When set, you should not share this handle between multiple threads. Otherwise, the result is undefined. |
Return value
UNQLITE_MEM Out of memory (Unlikely scenario).
Example
int rc;
unqlite *pDb;
// Open our database;
rc = unqlite_open(&pDb,"test.db",UNQLITE_OPEN_CREATE);
if( rc != UNQLITE_OK ){ return; }
//First data chunk .
rc = unqlite_kv_store(pDb,"msg",-1,"Hello, ",7); //msg => 'Hello, '
if( rc == UNQLITE_OK ){
//The second chunk
rc = unqlite_kv_append(pDb,"msg",-1,"Current time is: ",17); //msg => 'Hello, Current time is: '
if( rc == UNQLITE_OK ){
//The last formatted chunk
rc = unqlite_kv_append_fmt(pDb,"msg",-1,"%d:%d:%d",10,16,53); //msg => 'Hello, Current time is: 10:16:53'
}
}
if( rc != UNQLITE_OK ){
const char *zBuf;
int iLen;
/* Something goes wrong, extract database error log */
unqlite_config(pDb,UNQLITE_CONFIG_ERR_LOG,&zBuf,&iLen);
if( iLen > 0 ){
puts(zBuf);
}
if( rc != UNQLITE_BUSY && rc != UNQLITE_NOTIMPLEMENTED ){
/* Rollback */
unqlite_rollback(pDb);
}
}
//Auto-commit the transaction and close our handle
unqlite_close(pDb);
See also
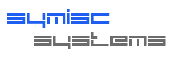