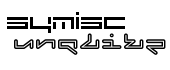
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
UnQLite C/C++ API Reference - Jx9 Script Compile.
int unqlite_compile(
unqlite *pDb,
const char *zJx9,
int nLen,
unqlite_vm **ppOutVm
);
Compile a Jx9 script to a bytecode program.
Description
To execute Jx9 code, it must first be compiled into a bytecode program using one of the compile interfaces. This routine takes as input a Jx9 script to compile and produce a bytecode program represented by an opaque pointer to the unqlite_vm structure. This routine does not actually evaluate the Jx9 code. It merely prepares it for later execution.
On successful compilation, the compiled program is stored in the ppOutVm pointer (Fourth parameter) and the program is ready for execution using unqlite_vm_exec(). When something goes wrong while compiling the Jx9 script due to a compile-time error, the caller must discard the ppOutVm pointer and fix its erroneous Jx9 code. The compile-time error log can be extracted (See example below) using the unqlite_config() interface with a configuration verb set to UNQLITE_CONFIG_JX9_ERR_LOG.
Parameters
pDb
|
A pointer to a unQLite database handle. |
zJx9
|
Jx9 script to compile. |
nLen
|
Jx9 script length. If the nLen argument is less than zero, then zJx9 is read up to the first zero terminator. If nLen is non-negative, then it is the maximum number of bytes read from zJx9. |
ppOutVm
|
OUT: On successful compilation, *ppOutVm is left pointing to the compiled Jx9 program represented by an opaque pointer to the unqlite_vm structure. It's safe now to call one of the VM management interfaces such as unqlite_vm_config() and the compiled program can be executed using unqlite_vm_exec(). Otherwise *ppOutVM is left pointing to NULL. |
Return value
UNQLITE_OK is returned on successful compilation of the target Jx9 script. Any other return value indicates failure such as:
UNQLITE_COMPILE_ERR is returned when compile-time errors occurs. That is, the caller must fix its erroneous Jx9 code and call the compile interface again.
Note that the compile-time error log can be extracted (See example below) using the unqlite_config() interface with a configuration verb set to:
UNQLITE_CONFIG_JX9_ERR_LOG
UNQLITE_VM_ERR is returned when something goes wrong during initialization of the virtual machine (i.e. Out of memory). But remember, this is a very unlikely scenario on modern hardware even on modern embedded system.
Example
Compile this C file for a smart introduction to this interface.
#define JX9_PROG \
"db_create('users'); /* Create the collection users */"\
" /* Store something */ "\
"db_store('users',{ 'name' : 'dean' , 'age' : 32 });"\
"db_store('users',{ 'name' : 'chems' , 'age' : 27 });"
int rc;
unqlite *pDb;
unqlite_vm *pVm;
// Open our database;
rc = unqlite_open(&pDb,"test.db",UNQLITE_OPEN_CREATE);
if( rc != UNQLITE_OK ){ return; }
// Compile our Jx9 program
rc = unqlite_compile(pDb,JX9_PROG,sizeof(JX9_PROG)-1,&pVm);
if( rc != UNQLITE_OK ){
/* Extract the compiler error-log */
const char *zBuf;
int iLen;
unqlite_config(pDb,UNQLITE_CONFIG_JX9_ERR_LOG,&zBuf,&iLen);
if( iLen > 0 ){
puts(zBuf);
}
return;
}
//Configure our VM ...
// Execute our Jx9 program
rc = unqlite_vm_exec(pVm);
if( rc != UNQLITE_OK ){ return; }
// Release our VM
unqlite_vm_release(pVm);
//Auto-commit the transaction and close our handle
unqlite_close(pDb);
See also
unqlite_vm_exec, unqlite_vm_config, unqlite_compile_file.
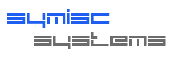