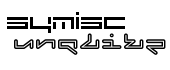
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
Jx9 Built-in Function - Database Storage & Retrieval Functions.
bool db_exists(string $collection_name);
Alias
collection_exists
Description
db_exists() return True if the given collection exists in the underlying database. If the collection does not exists, you can create a new one via db_create().
Parameters
$collection_name | Name of the target collection. |
Return Values
Example
$zCol = 'users'; /* Target collection name */
/* Check if the collection 'users' exists */
if( db_exists($zCol) ){
print "Collection users already created\n";
}else{
/* Try to create it */
$rc = db_create($zCol);
if ( !$rc ){
//Handle error db_errlog()
return;
}
print "Collection users successfully created\n";
}
//Store some JSON now db_store() ...
bool db_create(string $collection_name);
Alias
collection_create
Description
db_create() tries to create the given collection in the underlying database.
Parameters
$collection_name | Name of the collection to be created. |
Return Values
Example
$zCol = 'users'; /* Target collection name */
/* Check if the collection 'users' exists */
if( db_exists($zCol) ){
print "Collection users already created\n";
}else{
/* Try to create it */
$rc = db_create($zCol);
if ( !$rc ){
//Handle error db_errlog()
return;
}
print "Collection users successfully created\n";
}
//Store some JSON now db_store() ...
int64 db_last_record_id(string $collection_name);
Description
db_last_record_id() return the unique ID (64-bit integer) of the last inserted record in the collection. You can extract the unique ID of a stored JSON object via the special __id field which is appended automatically to each object after successful return from db_store().
Parameters
$collection_name | Name of the target collection. |
Return Values
int64 db_current_record_id(string $collection_name);
Description
db_current_record_id() return the unique ID (64-bit integer) of the record pointed by the internal cursor. You can also extract the unique ID of a stored JSON object via the special __id field which is appended automatically to each object after successful return from db_store().
Parameters
$collection_name | Name of the target collection. |
Return Values
bool db_reset_record_cursor(string $collection_name);
Description
db_reset_record_cursor() reset the internal record cursor so that a call to db_fetch() can re-start from the beginning.
Parameters
$collection_name | Name of the target collection. |
Return Values
int64 db_total_records(string $collection_name);
Description
Parameters
$collection_name | Name of the target collection. |
Return Values
string db_creation_date(string $collection_name);
Description
Parameters
$collection_name | Name of the target collection. |
Return Values
bool db_begin(void);
Description
Tip: For maximum concurrency, it is preferable to let UnQLite start the transaction for you automatically.
Return Values
bool db_commit(void);
Description
Tip: For maximum concurrency, it is recommended that you commit your transaction manually as soon as you have no more insertions.
Return Values
bool db_rollback(void);
Description
Return Values
bool db_drop_collection(string $collection_name);
Alias
collection_delete
Description
Parameters
$collection_name | Name of the target collection. |
Return Values
bool db_drop_record(string $collection_name, int64 $record_id);
Description
Parameters
$collection_name | Name of the target collection. |
$record_id |
Unique ID of the record to be deleted. |
Return Values
bool db_set_schema(string $collection_name, string $json_object_schema);
Description
Parameters
$collection_name | Name of the target collection. |
$json_object_schema |
Collection schema which must be a JSON object (See exmaple below). |
Return Values
Example
$zCol = 'users'; /* Target collection name */
/* Check if the collection 'users' exists */
if( db_exists($zCol) ){
print "Collection users already created\n";
}else{
/* Try to create it */
$rc = db_create($zCol);
if ( !$rc ){
//Handle error db_errlog()
return;
}
print "Collection users successfully created\n";
}
//Set a schema for our collection
$zSchema = {
name : 'string',
age : 'integer',
addr : 'string'
};
db_set_schema('users',$zSchema);
//Store some JSON now db_store() ...
object db_get_schema(string $collection_name);
Description
Parameters
$collection_name | Name of the target collection. |
Return Values
Collection schema (JSON object) is returned on success. NULL otherwise.
string db_version(void);
Description
Return Values
UnQLite database engine version.
string db_sig(void);
Description
Return Values
UnQLite database engine unique signature.
string db_copyright(void);
Alias
Description
Return Values
UnQLite copyright notice.
string db_errlog(void);
Description
Return Values
Database error log or the empty string.
Syntax
value db_fetch_by_id(string $collection_name,int64 $record_id);
Alias
db_get_by_id
Description
Fetch a record via its unique ID from the given collection. Note that you can extract the unique ID of a stored JSON object via the special __id field which is appended automatically to each object after successful return from db_store().
Parameters
$collection_name | Name of the target collection. |
$record_id |
Unique record ID |
Return Values
Example
/* Create the collection 'users' */
if( !db_exists('users') ){
/* Try to create it */
$rc = db_create('users');
if ( !$rc ){
//Handle error
print db_errlog();
return;
}
}
//Our simple JSON object to be stored shortly.
$doc = {name : 'james',
age : 28,
mail : 'dude@example.com',
};
//Perform the store operation in the collection users
$rc = db_store('users',$doc);
if( !$rc ){
//Handle error
print db_errlog();
return;
}/* Extract our record using its unique ID which is appended automatically by the engine.
*/
print db_fetch_by_id('users',$doc.__id);
//Remove our object via its unique ID
db_drop_record('users',$doc.__id);
Syntax
value db_fetch(string $collection_name);
Alias
db_get
Description
Fetch the current record from the target collection and automatically advance the cursor to the next record in the collection.
Note that you can manually reset the internal record cursor using db_reset_record_cursor().
Parameters
$collection_name | Name of the target collection. |
Return Values
Example
/* Create the collection 'users' */
if( !db_exists('users') ){
/* Try to create it */
$rc = db_create('users');
if ( !$rc ){
//Handle error
print db_errlog();
return;
}
}
//Store some JSON objects in our collection
db_store('users',{ name : 'robert', age : 35, mail : 'rob@example.com' } );
db_store('users',{ name : 'monji', age : 47, mail : 'monji@example.com' } );
db_store('users',{ name : 'barzini', age : 52, mail : 'barz@mobster.com' } );
//Fetch our records one after one from the collection
//db_reset_record_cursor();
while( ($rec = db_fetch('users')) != NULL ){
print $rec;
}
Syntax
array db_fetch_all(string $collection_name,callback $filter_callback);
Alias
db_get_all
Description
Retrieve all records from a given collection and apply the filter callback if available.
Parameters
$collection_name | Name of the target collection. |
$filter_callback |
Optional: Filter callback to apply to the retrieved records. The callback must accept one argument which is the current retrieved record passed verbatim by the engine to the callback. The callback must return a boolean result. TRUE when the record correspond to the callback criteria. FALSE otherwise (See example below). |
Return Values
JSON array holding the filtered records if any. NULL is returned on failure.
Example
/* Create the collection 'users' */
if( !db_exists('users') ){
/* Try to create it */
$rc = db_create('users');
if ( !$rc ){
//Handle error
print db_errlog();
return;
}
}
//The following is the records to be stored shortly in our collection
$zRec = [
{
name : 'james',
age : 27,
mail : 'dude@example.com'
},
{
name : 'robert',
age : 35,
mail : 'rob@example.com'
},
{
name : 'monji',
age : 47,
mail : 'monji@example.com'
},{
name : 'barzini',
age : 52,
mail : 'barz@mobster.com'
}
];
//Store our records
if( !$rc ){
//Handle error
print db_errlog();
return;
}//Create our filter callback
$zCallback = function($rec){
//Allow only users >= 30 years old.
if( $rec.age < 30 ){
// Discard this record
return FALSE;
}
//Record correspond to our criteria
return TRUE;
}; //Don't forget the semi-colon here
//Retrieve collection records and apply our filter callback
$data = db_fetch_all('users',$zCallback);
print $data; //JSON array holding the filtered records
Syntax
bool db_store(string $collection_name,$record,...);
Alias
db_put
Description
Store one or more JSON values (i.e. Objects, Arrays, Strings, etc.) in the given collection. The stored value is called collection record.
Parameters
$collection_name | Name of the target collection. |
$record |
One or more JSON values to be stored in the target collection (See example below). |
Return Values
TRUE is returned on successful insertion. FALSE otherwise.
Example
/* Create the collection 'users' */
if( !db_exists('users') ){
/* Try to create it */
$rc = db_create('users');
if ( !$rc ){
//Handle error
print db_errlog();
return;
}
}
//The following is the records to be stored shortly in our collection
$zRec = [
{
name : 'james',
age : 27,
mail : 'dude@example.com'
},
{
name : 'robert',
age : 35,
mail : 'rob@example.com'
},
{
name : 'monji',
age : 47,
mail : 'monji@example.com'
},{
name : 'barzini',
age : 52,
mail : 'barz@mobster.com'
}
];
//Store our records
if( !$rc ){
//Handle error
print db_errlog();
return;
}//One more record
$rc = db_store('users',{ name : 'alex', age : 19, mail : 'alex@example.com' });
if( !$rc ){
//Handle error
print db_errlog();
return;
}print "Total number of stored records: ",db_total_records('users');
//Fetch data using db_fetch_all(), db_fetch_by_id() and db_fetch().
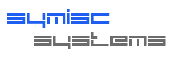