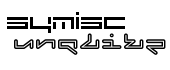
An Embeddable NoSQL Database Engine |
Star |
Follow @symisc |
Tweet |
Follow @unqlite_db |
Jx9 Samples.
Hello
world from Jx9
print "Hello World\n";
print "Host Operating System: ",JX9_OS,JX9_EOL; // Unix
print "Current date: ", __DATE__,JX9_EOL; // 2013-05-14
print "Current time: ", __TIME__; // 12:43:52
A more complex Hello world program.
$my_data = {
// Greeting message
greeting : "Hello world!\n",
// Host Operating System
os_name: uname(), //Built-in OS function
// Current date
date : __DATE__,
// Return the current time using an anonymous function
time : function(){ return __TIME__; }
};
// invoke the object fields
print $my_data.greeting; //Hello world!
print "Host Operating System: ", $my_data.os_name, JX9_EOL;
print "Current date: ", $my_data.date, JX9_EOL;
print "Current time: ", $my_data.time(); // Anonymous function
That is, this simple Jx9 script when running should display a greeting message, the current system time and the host operating system. A typical output of this program would look like this:
Host Operating System: Microsoft Windows 7 localhost 6.1 build 7600 x86
Current date: 2012-12-27
Current time: 10:36:02
Another Jx9 script which demonstrate how to use the built-in IO facility.
$num = [];
//Populate the JSON array
print "Enter a number (Decimal, Hex, Octal or Binary notation)\n";
$num[] = (int)fgets(STDIN);
print "Another number\n";
$num[] = (int)fgets(STDIN);
print "Finally, one last number\n";
$num[] = (int)fgets(STDIN);
//show content
print "Before sorting\n";
print $num;
//Sort the array now
sort($num);
print "\nAfter sorting\n";
print $num;
This simple Jx9 script ask for three numbers (64 bit integers) from the standard input (STDIN), store the given numbers in a JSON array, perform a merge-sort using the built-in sort function and output the sorted array. A typical output of this program would look like this:
50232
Another number
80
Finally, one last number
0xff
Before sorting
[50232,80,255]
After sorting
[80,255,50232]
An implementation of the famous factorial function in Jx9.
print "Enter a number\n";
$num = (int)fgets(STDIN);
function factorial(int $num)
{
if( $num == 0 || $num == 1 )
return 1;
else
return
$num
* factorial($num – 1);
}
print "Facorial of $num = ".. /* .. (Two dots) is the concatenation operator*/ factorial($num);
Another script which demonstrate how Jx9 can be used for processing external configuration file based on JSON using the built-in json_encode() and json_decode() functions.
// Create a dummy JSON object configuration
$my_config = {
bind_ip : "127.0.0.1",
config : "/etc/symisc/jx9.conf",
dbpath : "/usr/local/unqlite",
fork : true,
logappend : true,
logpath : "/var/log/jx9.log",
quiet : true,
port : 8080
};
// Dump the JSON object
print $my_config;
// Write to disk
$file = "config.json.txt";
print "\n\n------------------\nWriting JSON object to disk file: '$file'\n";
// Create the file
$fp = fopen($file,'w+');
if( !$fp )
exit("Cannot create $file");
// Write the JSON object
fwrite($fp,$my_config);
// Close the file
fclose($fp);
// All done
print "$file successfully created on: "..__DATE__..' '..__TIME__;
// ---------------------- config_reader.jx9 ----------------------------
// Read the configuration file defined above
$iSz = filesize($zFile); // Size of the whole file
$fp = fopen($zFile,'r'); // Read-only mode
if( !$fp ){
exit("Cannot open '$zFile'");
}
// Read the raw content
$zBuf = fread($fp,$iSz);
// decode the JSON object now
$my_config = json_decode($zBuf);
if( !$my_config ){
print "JSON decoding error\n";
}else{
// Dump the JSON object
foreach( $my_config as $key,$value ){
print "$key ===> $value\n";
}
}
// Close the file
fclose($fp);
The first script generate a JSON object holding some dummy configuration fields, create a disk file named config.json.txt using fopen() and serialize the JSON object to disk using fwrite() while the second script perform the reverse operation using the fread() and json_decode() functions.
And finally, a Jx9 script which store JSON objects (a collections of dummy users) in a UnQLite database.
/* Create the collection 'users' */
if( !db_exists('users') ){
/* Try to create it */
$rc = db_create('users');
if ( !$rc ){
//Handle error
print db_errlog();
return;
}
}
//The following is the records to be stored shortly in our collection
$zRec = [
{
name : 'james',
age : 27,
mail : 'dude@example.com'
},
{
name : 'robert',
age : 35,
mail : 'rob@example.com'
},
{
name : 'monji',
age : 47,
mail : 'monji@example.com'
},{
name : 'barzini',
age : 52,
mail : 'barz@mobster.com'
}
];
//Store our records
if( !$rc ){
//Handle error
print db_errlog();
return;
}//Create our filter callback
$zCallback = function($rec){
//Allow only users >= 30 years old.
if( $rec.age < 30 ){
// Discard this record
return FALSE;
}
//Record correspond to our criteria
return TRUE;
}; //Don't forget the semi-colon here
//Retrieve collection records and apply our filter callback
$data = db_fetch_all('users',$zCallback);
print $data; //JSON array holding the filtered records
As you can see, Jx9 is a powerful programming language with a friendly and familiar syntax built with a rich standard library with over 312 functions and 142 constants suitable for most purposes. Refer to the Jx9 Language Reference Manual for additional information. The Document store to UnQLite which is used to store JSON docs (i.e. Objects, Arrays, Strings, etc.) in the database is powered by the Jx9 programming language.
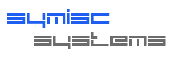