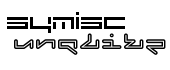
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
UnQLite C/C++ API Reference - Extracting Data From the Database.
unqlite *pDb,
const void *pKey,int nKeyLen,
void *pBuf,
unqlite_int64 *pSize /* IN: Buffer Size / OUT: Record Data Size */
);
Fetch a record from the database.
Description
Fetch a record from the database and copy its content to the user supplied buffer. This interface support both dynamically and statically allocated buffer (See example below).
The recommended interface for extracting very large data from the database is unqlite_kv_fetch_callback() where the user simply need to supply a consumer callback instead of a buffer which may be unacceptable when dealing with very large records.
Parameters
pDb
|
A pointer to a unQLite Database Handle. |
pKey
|
Record key. |
nKeyLen
|
pKey length. If the nKeyLen argument is less than zero, then pKey is read up to the first zero terminator. If nKeyLen is non-negative, then it is the maximum number of bytes read from pKey. |
pBuf
|
User supplied buffer where record content is copied in. |
pSize
|
IN: Supplied
buffer (pBuf) size in bytes.
OUT: Reccord data size in bytes or the amount of data copied in pBuf if the given buffer is too short. |
Return value
UNQLITE_NOTFOUND: Nonexistent record.
UNQLITE_BUSY: Another thread or process have an exclusive lock on the database. In this case, the caller should wait until the lock holder relinquish it.
UNQLITE_IOERR: OS specific error.
UNQLITE_NOMEM: Out of memory (Unlikely scenario).
For a human-readable error message, you can extract the database error log via unqlite_config() with a configuration verb set to UNQLITE_CONFIG_ERR_LOG.
Example
Compile this C file for a smart introduction to these interface.
Extract data using a dynamically allocated buffer.
unqlite *pDb;
size_t nBytes; //Data length
char *zBuf; //Dynamically allocated buffer
// Open our database;
rc = unqlite_open(&pDb,"test.db",UNQLITE_OPEN_READONLY|UNQLITE_OPEN_MMAP);
if( rc != UNQLITE_OK ){ return; }
//Extract data size first
rc = unqlite_kv_fetch(pDb,"record",-1,NULL,&nBytes);
if( rc != UNQLITE_OK ){
return;
}
//Allocate a buffer big enough to hold the record content
zBuf = (char *)malloc(nBytes);
if( zBuf == NULL ){ return; }
//Copy record content in our buffer
unqlite_kv_fetch(pDb,"record",-1,zBuf,&nBytes);
//Play with zBuf...
free(zBuf);
//Close our database handle
unqlite_close(pDb);
Extract data using a statically allocated buffer.
#define MAX_BUF 1024 /* Static buffer size */
unqlite *pDb;
size_t nBytes; //Data length
char zBuf[MAX_BUF]; //Static buffer
// Open our database;
rc = unqlite_open(&pDb,"test.db",UNQLITE_OPEN_READONLY|UNQLITE_OPEN_MMAP);
if( rc != UNQLITE_OK ){ return; }
//Extract record content
nBytes = MAX_BUF;
rc = unqlite_kv_fetch(pDb,"record",-1,zBuf,&nBytes);
if( rc != UNQLITE_OK ){
return;
}
//Play with zBuf...
//Close our database handle
unqlite_close(pDb);
See also
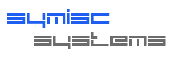