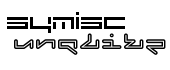
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
UnQLite C/C++ API Reference - Extracting Data From the Database.
unqlite *pDb,
const void *pKey,int nKeyLen,
int (*xConsumer)(const void *pData,unsigned int iDataLen,void *pUserData),
void *pUserData
);
Fetch a record from the database and invoke the supplied callback to consume its data.
Description
This is the recommended interface to use by clients for data extraction. Rather than allocating a static or dynamic buffer (Inefficient scenario for large data), the caller simply need to supply a consumer callback which is responsible of consuming the record data perhaps redirecting it (i.e. Record data) to its standard output (STDOUT), disk file, connected peer and so forth (See example below).
Depending on how large the extracted data, the callback may be invoked more than once.
Parameters
pDb
|
A pointer to a unQLite Database Handle. |
pKey
|
Record key. |
nKeyLen
|
pKey length. If the nKeyLen argument is less than zero, then pKey is read up to the first zero terminator. If nKeyLen is non-negative, then it is the maximum number of bytes read from pKey. |
int (*xConsumer)(
const void *pData, unsigned int iDataLen, void *pUserData ) |
Data Consumer callback. The callback must accept three arguments:
The first argument is a pointer to the extracted data chunk.
The
second argument is the length in bytes of the extracted chunk. The last argument is a copy of the pointer (callback private data) passed as the fifth argument to this routine. When done, the callback must return UNQLITE_OK. But, if for some reason the callback wishes to abort processing and thus to stop its invocation, it must return UNQLITE_ABORT instead.
|
pUserData
|
Arbitrary user pointer which is forwarded verbatim by the engine to the callback as its third argument. |
Return value
UNQLITE_NOTFOUND: Nonexistent record.
UNQLITE_BUSY: Another thread or process have an exclusive lock on the database. In this case, the caller should wait until the lock holder relinquish it.
UNQLITE_IOERR: OS specific error.
UNQLITE_NOMEM: Out of memory (An unlikely scenario).
UNQLITE_ABORT: Client consumer callback request an operation abort.
For a human-readable error message, you can extract the database error log via unqlite_config() with a configuration verb set to UNQLITE_CONFIG_ERR_LOG.
Example
Compile this C file for a smart introduction to these interface.
#include <unqlite.h>
/* A consumer callback which redirect the record data to its standard output (STDOUT) */
static int DataConsumerCallback1(const void *pOutput,unsigned int nOutputLen,void *pUserData)
{
ssize_t nWr;
nWr = write(STDOUT_FILENO,pOutput,nOutputLen);
if( nWr < 0 ){
/* Abort processing */
return UNQLITE_ABORT;
}
/* All done, data was redirected to STDOUT */
return UNQLITE_OK;
}
/* Another data consumer callback which redirect the record data to a connected client */
static int DataConsumerCallback2(const void *pOutput,unsigned int nOutputLen,void *pUserData)
{
conn_t *pConn = (conn_t *)pUserData; /* Callback private data */
ssize_t nWr;
/* Send data to the peer */
nWr = write(pConn->cli_fd,pOutput,nOutputLen);
if( nWr < 0 ){
/* Peer hangs on us, abort processing */
return UNQLITE_ABORT;
}
/* All done, data is sent to the peer */
return UNQLITE_OK;
}
{
int rc;
unqlite *pDb;
// Open our database;
rc = unqlite_open(&pDb,"test.db",UNQLITE_OPEN_READONLY|UNQLITE_OPEN_MMAP);
if( rc != UNQLITE_OK ){ return; }
//Fetch our records and invoke the supplied callbacks
unqlite_kv_fetch_callback(&pDb,"MyRecord",-1,DataConsumerCallback1,NULL/* Callback private data */);
unqlite_kv_fetch_callback(&pDb,"OtherRecord",-1,DataConsumerCallback2,newConn(fd)/* Callback private data */);
//Close our database handle
unqlite_close(pDb);
}
See also
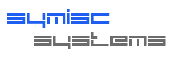