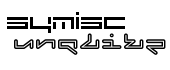
An Embeddable NoSQL Database Engine |
Tweet |
Follow @unqlite_db |
UnQLite C/C++ API Reference - Delete Database Record.
int unqlite_kv_delete(unqlite *pDb,const void *pKey,int nKeyLen);
Remove a record from the database.
Description
To remove a particular record from the database, you can use this high-level thread-safe routine to perform the deletion. You can also delete records using cursors via unqlite_kv_cursor_delete_entry().
Parameters
pDb
|
A pointer to a unQLite Database Handle. |
pKey
|
Target record key. |
nKeyLen
|
pKey length. If the nKeyLen argument is less than zero, then pKey is read up to the first zero terminator. If nKeyLen is non-negative, then it is the maximum number of bytes read from pKey. |
Return value
UNQLITE_NOTFOUND: Nonexistent record.
UNQLITE_BUSY: Another thread or process have a reserved or exclusive lock on the database. In which case, the caller should wait until the lock holder relinquish it.
UNQLITE_READ_ONLY: Read-only Key/Value storage engine.
UNQLITE_NOTIMPLEMENTED: The underlying KV storage engine does not implement the delete operation.
UNQLITE_PERM: Permission error.
UNQLITE_LIMIT: Journal file record limit reached (An unlikely scenario).
UNQLITE_IOERR: OS specific error. This error is considered harmful and you should perform an immediate rollback via unqlite_rollback().
UNQLITE_NOMEM: Out of memory (Unlikely scenario). This error is considered harmful and you should perform an immediate rollback via unqlite_rollback().
For a human-readable error message, you can extract the database error log via unqlite_config() with a configuration verb set to UNQLITE_CONFIG_ERR_LOG.
Example
int rc;
unqlite *pDb;
// Open our database;
rc = unqlite_open(&pDb,"test.db",UNQLITE_OPEN_READWRITE);
if( rc != UNQLITE_OK ){ return; }
//Store some records unqlite_kv_append, unqlite_kv_store...
//Delete a record
rc = unqlite_kv_delete(pDb,"SomeRecord",-1);
if( rc != UNQLITE_OK ){
const char *zBuf;
int iLen;
/* Extract database error log */
unqlite_config(pDb,UNQLITE_CONFIG_ERR_LOG,&zBuf,&iLen);
if( iLen > 0 ){
puts(zBuf);
}
if( rc != UNQLITE_BUSY && rc != UNQLITE_NOTFOUND ){
/* Rollback */
unqlite_rollback(pDb);
}
}
//Auto-commit the transaction and close our handle
unqlite_close(pDb);
See also
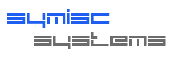